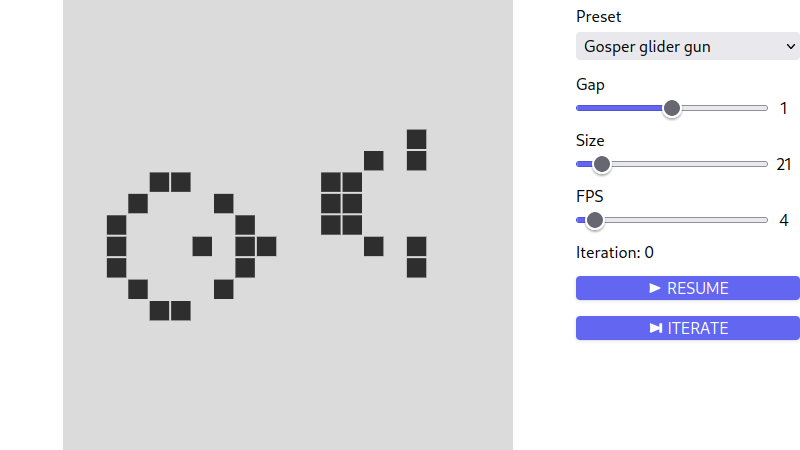
Click here to try it
libre_game_of_life
FLOSS Implementation of Conway’s Game Of Life
The Game of Life is a cellular automaton devised by the british mathematician John Horton Conway in 1970
Rules
Game of Life automaton occurs on a grid where each cell can be either dead or alive. At each time step, what determines the state of each cell are the following rules:
- Alive cells survive with 2 or 3 alive neighbors
- Dead cells become alive with 3 alive neighbors
My implementation
I implemented a version of Game of Life in Rust.
let grid = HashMap::from([
(Coordinate::from(0, 1), State::Alive),
(Coordinate::from(-4, 2), State::Alive),
(Coordinate::from(7, 3), State::Alive),
]);
In the code above, the implementation of the automaton grid. A HashSet would also work in this scenario, because only alive cells are considered. However, some variations of Game of Life have more states
When using a HashMap, we decouple the grid and the render, allowing us to render any part of the grid, zooming in and out, without affecting the current state.
Another aspect is that, we need some coordinate system to identify a specific position. The most simple is the cartesian plane, which is the one used.
Iterating
To create the next generation, the current HashMap is traversed, and for each alive cell and its neighbors, the rules are applied. The resulting alive cells are saved in a new HashMap.
The application
I implemented Game of Life on the web, rendered on a canvas. The project has three layers:
lib
A reusable, generic implementation of Game of Life in Rust.
web_backend
A Web Assembly application that works as a bridge between lib and web_frontend.
web_frontend
The user application, responsible for render the canvas, the settings, and init the Web Assembly. Currently, the settings are the following:
- Preset: Allows the user to choose from many popular shapes (Glider, Blinker, etc.)
- Gap: A visual option, for aesthetics
- Size: The amount of zoom in the screen
- FPS: The desired FPS for render
Reference
https://en.wikipedia.org/wiki/Conway%27s_Game_of_Life
Published: 2023-05-07
Updated: 2025-02-12
License: GNU AGPLv3
Repository: https://github.com/joao-arthur/libre_game_of_life
Programming languages: Rust, TypeScript
Technologies: Web Assembly, Svelte